Receive your feedback reports as JSON, POSTed to a webhook url you specify.
How to set up
Configuration is per-project in Ybug. To set up forwarding of your feedback reports to a Webhook, go to your dashboard and follow these simple steps:
1. Go to Integrations tab in your project settings.
2. Click the On/Off switch in the Webhook row.
3. Enter your webhook url. You can test the integration by clicking the Test button. When done, do not forget to click the Save settings button.
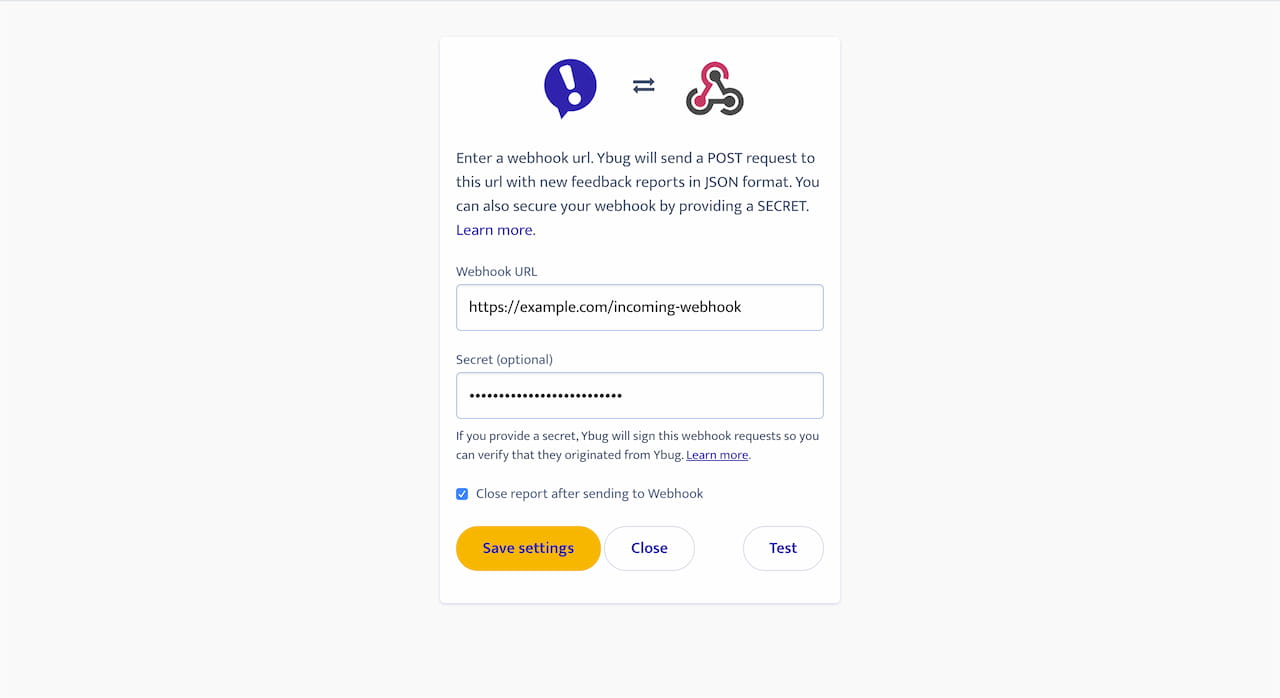
Securing your webhook
Webhooks deliver data to publicly accessible URLs, it is a good idea to secure your data and your endpoint. You can provide a secret
value while setting up the webhook integration. Ybug will then use your secret
to sign all the webhook requests so you can verify that they originated from Ybug.
Each request will contain a HTTP header X-Ybug-Signature
, which is a HMAC hex digest of the request body generated using the sha256
hash function and the secret
as the HMAC key.
X-Ybug-Signature header looks something like this (with an actual hash instead of Xs):
X-Ybug-Signature: sha256=XXXXXXXXXXXXXXXXXXX
Generating a secret
You can use any random string generator to create a webhook secret. It should be at least 32 characters long. You can for example use php to generate your secret:
php -r 'echo bin2hex(random_bytes(32));'
How to verify the signature?
To verify the signature in the X-Ybug-Signature
header, you need to compute the HMAC hex digest using
the same algorithm (sha256
), your secret
key and the request body and ensure
that your computed hash matches the signature from Ybug.
Your code then could look like this (in PHP):
// X-Ybug-Signature header contains sha256=XXXXXXXXXXXXXXXXXXX
// Explode it to get the algo=sha256 and signature=XXXXXXXXXXXXXXXXXXX
list($algo, $signature) = explode('=', $reqHeaders['X-Ybug-Signature']);
// Compute hmac digest
// $reqPayload should contain the raw request body, something like {"id":"t3str3p0rt","title":"Sample feedback report","reportId":0,...
$signature2 = hash_hmac($algo, $reqPayload, MY_WEBHOOK_SECRET);
// Verify the request signature (do not use ==/=== string comparison here, as it is not safe against timing attacks)
// Use hash_equals in PHP or similar in other languages
if (!hash_equals($signature2, $signature)) {
throw new Exception('Ybug signature verification failed');
}
// Signature was verified
// ... do something with the payload
Responding to a webhook
To acknowledge that you received our webhook request, your endpoint should return a 2xx HTTP status code. Any other HTTP code will indicate to Ybug that you didn't receive the webhook.
Retry policy
If the sent webhook request fails, Ybug will try to resend it up to 5 times. Each subsequent retry is delayed with an exponential back-off algorithm.
How to unsubscribe your webhook
You can turn off your webhook subscription either in your dashboard or by responding with 410 Gone
status code.
Feedback report JSON payload
{
"id": "t3str3p0rt",
"title": "Sample feedback report",
"reportId": 1,
"projectId": "t3stpr0j3ctkey",
"reporter": {
"name": "Tester",
"email": "[email protected]",
"phone": null
},
"url": "https:\/\/www.example.com",
"comment": "Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Integer malesuada. Maecenas lorem. Vestibulum fermentum tortor id mi. Integer rutrum, orci vestibulum ullamcorper ultricies, lacus quam ultricies odio, vitae placerat pede sem sit amet enim. Nullam rhoncus aliquam metus. Nam quis nulla. Suspendisse nisl.",
"rating": 5,
"ratingType": "stars",
"netPromoterScore": null,
"priority": {
"id": "2",
"name": "High"
},
"type": {
"id": "1",
"name": "Bug"
},
"sourceType": "widget",
"createdAt": "2019-11-10T06:58:01Z",
"updatedAt": "2023-03-11T09:05:56Z",
"attributes": {
"screenWidth": "1280",
"screenHeight": "800",
"screenPixelDepth": "24",
"browser": "Safari",
"platform": "macOS",
"screenPixelRatio": "1",
"browserVersion": "12.1.1",
"deviceType": "desktop",
"viewportWidth": "1280",
"viewportHeight": "739",
"platformVersion": "10.14.5",
"consoleLog": "{\"error\":3,\"info\":2}",
"referrer": "https:\/\/referrer.com",
"location": "US, California, San Francisco"
},
"customUserData": "{\"name\":\"Tester\",\"session\":\"MYSESSIONID\"}",
"reportUrl": "http:\/\/ybug-dev.io\/dashboard\/reports\/detail\/t3str3p0rt",
"screenshotUrl": "http:\/\/ybug-dev.io\/data\/reports\/tstproj3ct\/t3str3p0rt\/screenshot.jpg?_token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpYXQiOjE3MDkxNTQ1NjYsImRhdGEiOnsicmVwb3J0IjoidDNzdHIzcDBydCIsInVzZXIiOiJBUEkifX0.e-ChzS4Gw1p0-FKMVLzHUlLNk9ok2qBEg0dBI2mcjnc",
"videoUrl": null,
"customFields": [
{
"id": "018a094b-6d42-71e2-bad6-1849d6be094a",
"name": "Example dropdown field",
"value": {
"id": "090f9131-dffe-435e-a2af-d37a517babb9",
"name": "Example option"
}
},
{
"id": "018c2ba6-0d61-7d20-a9cb-0b7300294445",
"name": "Example text field",
"value": "Lorem ipsum dolor sit amet!"
},
{
"id": "018df17b-ed91-7e04-800c-1b8c3d1411ad",
"name": "Example date field",
"value": "2024-02-29"
},
{
"id": "018df17c-0705-725a-8628-aab120617437",
"name": "Example number field",
"value": 1234
},
{
"id": "018df17c-2fec-7c2d-86c1-3041caea39fa",
"name": "Example checkbox field",
"value": true
}
]
}
Field specifications
Field | Type | Values |
---|---|---|
priority | null|object | Object with id and name. Possible values: 1 = Highest, 2 = High, 3 = Medium, 4 = Low, 5 = Lowest |
type | null|object | Object with id and name. Possible values: 1 = Bug, 2 = Improvement, 3 = Question, 4 = Feedback |
rating | null|integer | Rating scale 1..5 (1 = Lowest ... 5 = Highest) |
ratingType | null|string | Rating type. Possible values: 'stars', 'hearts', 'emoji' |